Vue Bootstrap Animations
Vue Animations - Bootstrap 4 & Material Design
Note: This documentation is for an older version of Bootstrap (v.4). A
newer version is available for Bootstrap 5. We recommend migrating to the latest version of our product - Material Design for
Bootstrap 5.
Go to docs v.5
Vue Bootstrap animations are illusions of motions for web elements. +70 animations generated by CSS only, work properly on every browser.
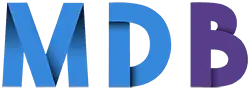
Basic usage
Using our animation is simple.
Step 1: Add the class .animated
to the element you want to animate.
Step 2: Add one of the following classes:
.bounce
.flash
.pulse
.rubberBand
.shake
.headShake
.swing
.tada
.wobble
.jello
.bounceIn
.bounceInDown
.bounceInLeft
.bounceInRight
.bounceInUp
.bounceOut
.bounceOutDown
.bounceOutLeft
.bounceOutRight
.bounceOutUp
.fadeIn
.fadeInDown
.fadeInDownBig
.fadeInLeft
.fadeInLeftBig
.fadeInRight
.fadeInRightBig
.fadeInUp
.fadeInUpBig
.fadeOut
.fadeOutDown
.fadeOutDownBig
.fadeOutLeft
.fadeOutLeftBig
.fadeOutRight
.fadeOutRightBig
.fadeOutUp
.fadeOutUpBig
.flipInX
.flipInY
.flipOutX
.flipOutY
.lightSpeedIn
.lightSpeedOut
.rotateIn
.rotateInDownLeft
.rotateInDownRight
.rotateInUpLeft
.rotateInUpRight
.rotateOut
.rotateOutDownLeft
.rotateOutDownRight
.rotateOutUpLeft
.rotateOutUpRight
.hinge
.rollIn
.rollOut
.zoomIn
.zoomInDown
.zoomInLeft
.zoomInRight
.zoomInUp
.zoomOut
.zoomOutDown
.zoomOutLeft
.zoomOutRight
.zoomOutUp
.slideInDown
.slideInLeft
.slideInRight
.slideInUp
.slideOutDown
.slideOutLeft
.slideOutRight
.slideOutUp
Step 3 (additionally): You may also want to include the class infinite for an infinite loop.
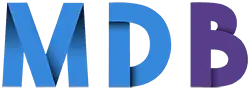
<template>
<img class="animated bounce infinite" src="https://mdbootstrap.com/img/logo/mdb-transparent.webp">
</template>
Reveal Animations When Scrolling
Thanks to MDB you can easily launch an animation on page scroll - as soon as element is fully visible on the screen
.webp)
.webp)
.webp)
.webp)
.webp)
.webp)
<template>
<section>
<div class="mb-5">
<mdb-row class="mb-4">
<mdb-col>
<img
src="https://mdbootstrap.com/img/Photos/Horizontal/Nature/4-col/img%20(31).webp"
alt="Sample image"
class="img-fluid"
v-animateOnScroll="{animation: 'bounceInLeft', delay: 300, position: 20}"
>
</mdb-col>
<mdb-col>
<img
src="https://mdbootstrap.com/img/Photos/Horizontal/Nature/4-col/img%20(32).webp"
alt="Sample image"
class="img-fluid"
v-animateOnScroll="'tada'"
>
</mdb-col>
<mdb-col>
<img
src="https://mdbootstrap.com/img/Photos/Horizontal/Nature/4-col/img%20(73).webp"
alt="Sample image"
class="img-fluid"
v-animateOnScroll="{animation: 'fadeInLeft', delay: 200}"
>
</mdb-col>
</mdb-row>
<mdb-row class="mb-4">
<mdb-col>
<img
src="https://mdbootstrap.com/img/Photos/Horizontal/Nature/4-col/img%20(34).webp"
alt="Sample image"
class="img-fluid"
v-animateOnScroll="'fadeInRight'"
>
</mdb-col>
<mdb-col>
<img
src="https://mdbootstrap.com/img/Photos/Horizontal/Nature/4-col/img%20(14).webp"
alt="Sample image"
class="img-fluid"
v-animateOnScroll="'fadeIn'"
>
</mdb-col>
<mdb-col>
<img
src="https://mdbootstrap.com/img/Photos/Horizontal/Nature/4-col/img%20(35).webp"
alt="Sample image"
class="img-fluid"
v-animateOnScroll="'rollIn'"
>
</mdb-col>
</mdb-row>
</div>
</section>
</template>
<script>
import { mdbRow, mdbCol, animateOnScroll } from 'mdbvue';
export default {
name: 'AnimationsPage',
components: {
mdbRow,
mdbCol,
},
directives: {
animateOnScroll
}
}
</script>
Basic usage
Step 1: Import animateOnScroll directive from 'mdbvue'
<script>
import { animateOnScroll } from "mdbvue";
</script>
Step 2: Add animateOnScroll to directives
<script>
import { animateOnScroll } from "mdbvue";
export default {
name: "AnimationsPage",
directives: {
animateOnScroll
}
}
</script>
Step 3: Pick an animation style from the list of animations and set the directive equal to its name:
<template>
<img
src="https://mdbootstrap.com/img/logo/mdb-transparent-250px.webp"
alt="Transparent MDB Logo"
v-animateOnScroll="'fadeIn'"
>
</template>
<script>
import { animateOnScroll } from "mdbvue";
export default {
name: "AnimationsPage",
directives: {
animateOnScroll
}
}
</script>
Step 4: Customize your animation according to your needs by passing an object to a v-animateOnScroll directive. Apart from animation class you can specify delay or position - delay takes time as an argument (in miliseconds) while position is an additional percent of a view port height user has to scroll before an animation starts.
<template>
<img
src="https://mdbootstrap.com/img/logo/mdb-transparent-250px.webp"
alt="Transparent MDB Logo"
v-animateOnScroll="{animation: 'fadeInRight', delay: 100, position: 12}"
>
</template>
<script>
import { animateOnScroll } from "mdbvue";
export default {
name: "AnimationsPage",
directives: {
animateOnScroll
}
}
</script>