Drag and drop / Draggable
Bootstrap drag and drop / draggable plugin
Note: We are transitioning MDB4 to a legacy version and focusing on developing MDB5.
While we'll continue to support for the transition period, we encourage you to migrate to
MDB5. We're offering a huge discount on MDB5 PRO to help with your transition,
enabling you to leverage the full potential of the latest version. You can find more information here.
upgrade with discount
MD Bootstrap's Draggable plugin is an extension that allows you to move objects by clicking on them and dragging them anywhere within the viewport.
To start working with the Draggable plugin, see the - "Getting Started" tab on this page.
Basic example
Draggable panel
I'm a draggable panel
<div id="draggable" class="card w-25">
<h5 class="card-header primary-color white-text">Draggable panel</h5>
<div class="card-body">
<p class="card-text">I'm draggable panel</p>
</div>
</div>
$('#draggable').draggable();
Auto-scroll
Use the scroll: true
option to enable auto-scrolling when an element is moved beyond its container
boundaries. It's also possible to change scroll sensitivity and speed with scrollSensitivity
and scrollSpeed
options.
Draggable panel
Drag me outside container boundary to enable auto-scroll
<div class="row">
<div class="col-md-4 col-6">
<div id="draggable-scroll" class="card">
<h5 class="card-header success-color white-text">Draggable panel</h5>
<div class="card-body">
<p class="card-text">Drag me outside container boundary to enable auto-scroll</p>
</div>
</div>
</div>
</div>
$('#draggable-scroll').draggable({
scroll: true,
scrollSensitivity: 40,
scrollSpeed: 40
});
Axis
You can limit a draggable element movement to one axis by setting either the axis: "x"
or axis: "y"
option.
Draggable panel
I can move only in the x-axis
Draggable panel
I can move only in the y-axis
<div class="row">
<div class="col-md-4 col-6">
<div id="draggable-x" class="card">
<h5 class="card-header primary-color white-text">Draggable panel</h5>
<div class="card-body">
<p class="card-text">I can move only in the x-axis </p>
</div>
</div>
</div>
<div class="col-md-4 col-6">
<div id="draggable-y" class="card">
<h5 class="card-header deep-orange white-text">Draggable panel</h5>
<div class="card-body">
<p class="card-text">I can move only in the y-axis</p>
</div>
</div>
</div>
</div>
$('#draggable-x').draggable({
axis: "x"
});
$('#draggable-y').draggable({
axis: "y"
});
Cursor style and position
By default, the cursor appears in the center of the dragged element. You can change the cursor style and position by
specifing the cursor
and cursorAt
options.
Draggable panel
I have a 'move' cursor positioned in the center
Draggable panel
I have a pointer cursor positioned in the top left corner
Draggable panel
I have a crosshair cursor positioned on the bottom
<div class="row">
<div class="col-md-4">
<div id="draggable-move" class="card">
<h5 class="card-header primary-color white-text">Draggable panel</h5>
<div class="card-body">
<p class="card-text">I have a 'move' cursor positioned in the center</p>
</div>
</div>
</div>
<div class="col-md-4">
<div id="draggable-pointer" class="card">
<h5 class="card-header deep-orange white-text">Draggable panel</h5>
<div class="card-body">
<p class="card-text">I have a pointer cursor positioned in the top left corner</p>
</div>
</div>
</div>
<div class="col-md-4">
<div id="draggable-crosshair" class="card">
<h5 class="card-header success-color white-text">Draggable panel</h5>
<div class="card-body">
<p class="card-text">I have a crosshair cursor positioned on the bottom</p>
</div>
</div>
</div>
</div>
$('#draggable-move').draggable({
cursor: "move"
});
$('#draggable-pointer').draggable({
cursor: "pointer",
cursorAt: { top: -5, left: -5 }
});
$('#draggable-crosshair').draggable({
cursor: "crosshair",
cursorAt: { bottom: 0 }
});
Handles
You can use the handle
and cancel
options to enable dragging only when the cursor is over a
specific part of the draggable element. In the following example you can drag the card around only when the mouse cursor is
over the card image.
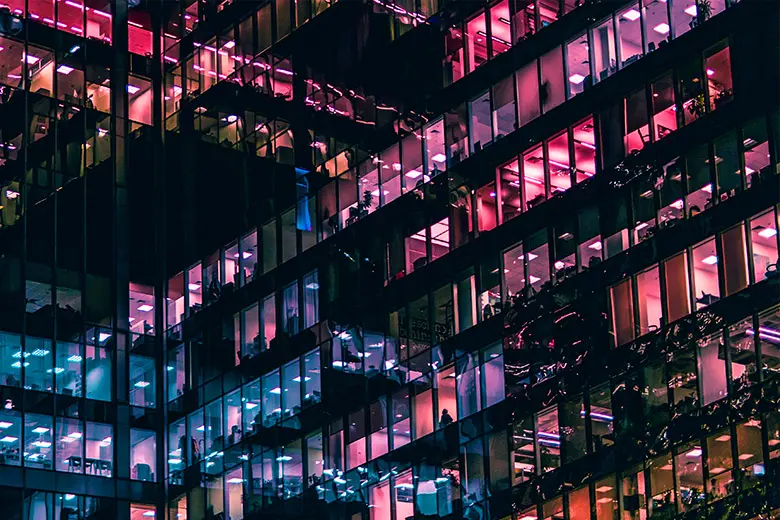
<div class="row">
<div class="col-md-3">
<!-- Card -->
<div id="draggable-handle" class="card">
<!-- Card image -->
<img class="card-img-top" src="https://mdbootstrap.com/img/Photos/Others/images/43.webp" alt="Card image cap">
<!-- Card content -->
<div class="card-body">
<!-- Title -->
<h4 class="card-title"><a>Draggable card</a></h4>
<!-- Text -->
<p class="card-text">Move the mouse over the image to drag</p>
<!-- Button -->
<a href="#" class="btn btn-primary">Button</a>
</div>
</div>
<!-- Card -->
</div>
</div>
$('#draggable-handle').draggable({
handle: '.card-img-top'
});
// or
$('#draggable-handle').draggable({
cancel: '.card-body'
});
Events
There are several events that are fired when you drag en element. Start
is fired at the start of the drag,
drag
during the dragging process, and stop
when dragging stops. You can specify callback
functions for those events as options.
.webp)
<div class="row">
<div class="col-md-4">
<!-- Card -->
<div id="draggable-events" class="card">
<!-- Card image -->
<img class="card-img-top" src="https://mdbootstrap.com/img/Photos/Lightbox/Thumbnail/img%20(147).webp" alt="Card image cap">
<!-- Card content -->
<div class="card-body">
<!-- Title -->
<h4 class="card-title"><a>Draggable card</a></h4>
<!-- Text -->
<p class="card-text">Start event fired <span class="start-event">0</span> times</p>
<hr>
<p class="card-text">Drag event fired <span class="drag-event">0</span> times</p>
<hr>
<p class="card-text">Stop event fired <span class="stop-event">0</span> times</p>
</div>
</div>
<!-- Card -->
</div>
</div>
$('#draggable-events').draggable({
start: onDragStart,
drag: onDrag,
stop: onDragStop
});
var $startCounter = $('.start-event');
var $dragCounter = $('.drag-event');
var $stopCounter = $('.stop-event');
var counts = {
start: 0,
drag: 0,
stop: 0
};
function onDragStart() {
counts.start++;
updateCounter($startCounter, counts.start);
}
function onDrag() {
counts.drag++;
updateCounter($dragCounter, counts.drag);
}
function onDragStop() {
counts.stop++;
updateCounter($stopCounter, counts.stop);
}
function updateCounter(selector, value) {
$(selector).text(value);
}
Snap to element
Use the snap
option to snap the draggable element to another DOM element and the snapTolerance
option to define the distance in pixels the draggable element must be from another element to enable snapping. There are three snap modes:
inner, outer, both.
Draggable panel
I will snap to the orange panel's outer edges
Draggable panel
I will snap to the body from a distance of 50px
<div class="container">
<div class="row">
<div class="col-md-4 col-6">
<div id="draggable-snap-1" class="card">
<h5 class="card-header primary-color white-text">Draggable panel</h5>
<div class="card-body">
<p class="card-text">I will snap to the orange panel's outer edges</p>
</div>
</div>
</div>
<div class="col-md-4 col-6">
<div id="draggable-snap-2" class="card">
<h5 class="card-header deep-orange white-text">Draggable panel</h5>
<div class="card-body">
<p class="card-text">I will snap to the body from a distance of 50px</p>
</div>
</div>
</div>
</div>
</div>
$('#draggable-snap-1').draggable({
snap: '#draggable-snap-2',
snapMode: 'outer'
});
$('#draggable-snap-2').draggable({
snap: 'body',
snapTolerance: '50'
});
Revert position
When the revert
option is enabled, draggable element is returned to its original position after drag stops.
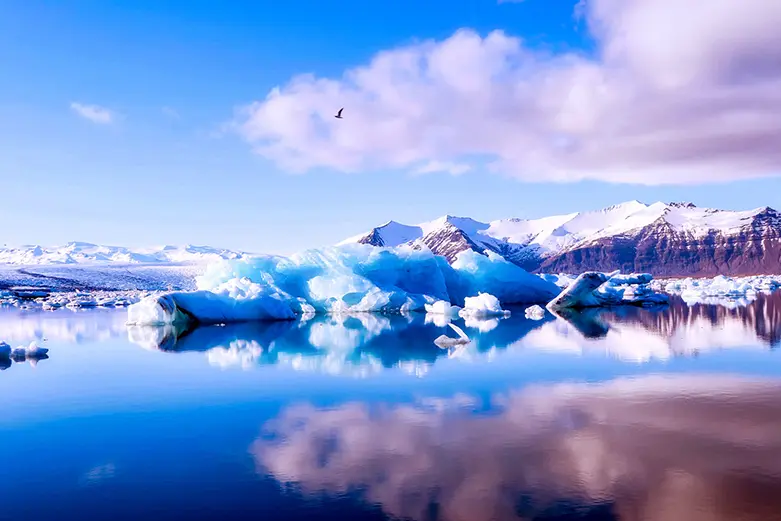
Draggable card
Revert original card
<div class="container">
<div class="row">
<div class="col-md-3">
<!-- Card -->
<div id="draggable-revert" class="card">
<!-- Card image -->
<img class="card-img-top" src="https://mdbootstrap.com/img/Photos/Others/images/49.webp" alt="Card image cap">
<!-- Card content -->
<div class="card-body">
<!-- Title -->
<h4 class="card-title"><a>Draggable card</a></h4>
<!-- Text -->
<p>Revert original card</p>
</div>
</div>
<!-- Card -->
</div>
$('#draggable-revert').draggable({
revert: true
});
Limit draggable movement
You can set the boundaries in which draggable elements can move by using the containment
option.
Draggable panel
I can only move inside the blue container
<div class="container" style="height: 1000px">
<div class="row h-100">
<div class="col-md-6 blue draggable-container">
<div class="row">
<div class="col-md-6">
<div id="draggable-containment" class="card card-body">
<h4 class="card-title">Draggable panel</h4>
<p class="card-text">I can only move inside the blue container</p>
</div>
</div>
</div>
</div>
<div class="col-md-6 mdb-color"></div>
</div>
$('#draggable-containment').draggable({
containment: '.draggable-container',
});
Drag and drop / Draggable - getting started : download & setup
Download
This plugin requires a purchase.
Buy Draggable plugin