React Bootstrap Images
React Images - Bootstrap 4 & Material Design
Note: This documentation is for an older version of Bootstrap (v.4). A
newer version is available for Bootstrap 5. We recommend migrating to the latest version of our product - Material Design for
Bootstrap 5.
Go to docs v.5
React Bootstrap images are automatically adjusted to all screen sizes - image will never be larger than parent elements. Its styles can be changed via classes.
Responsive images
import React from "react";
import { MDBContainer, MDBRow, MDBCol } from "mdbreact";
class MasksPage extends React.Component {
render() {
return (
<MDBContainer className="mt-5">
<MDBRow className="mb-4">
<MDBCol md="4">
<img src="https://mdbootstrap.com/img/Others/documentation/1.webp" className="img-fluid" alt="" />
</MDBCol>
</MDBRow>
<MDBRow className="mb-4">
<MDBCol md="6">
<img src="https://mdbootstrap.com/img/Others/documentation/1.webp" className="img-fluid" alt="" />
</MDBCol>
</MDBRow>
<MDBRow className="mb-4">
<MDBCol md="8">
<img src="https://mdbootstrap.com/img/Others/documentation/1.webp" className="img-fluid" alt="" />
</MDBCol>
</MDBRow>
</MDBContainer>
);
}
}
export default MasksPage;
SVG images and IE 10
In Internet Explorer 10, SVG images with
.img-fluid
are disproportionately sized. To fix this, addwidth: 100% \9;
where necessary. This fix improperly sizes other image formats, so Bootstrap doesn’t apply it automatically.
Image thumbnails
In addition to our border-radius
utilities, you can use .img-thumbnail
to give an image a rounded 1px border appearance.
<MDBRow>
<MDBCol>
<img src="https://mdbootstrap.com/img/Others/documentation/img%20(75)-mini.webp" alt="thumbnail" className="img-thumbnail" />
</MDBCol>
</MDBRow>
Aligning Images
Align images with the helper
float classes or text alignment
classes
. block
-level images can be centered using the
.mx-auto margin utility class.
<MDBRow>
<MDBCol>
<img src="https://mdbootstrap.com/img/Photos/Avatars/avatar-1.webp" className="rounded float-left" alt="aligment" />
<img src="https://mdbootstrap.com/img/Photos/Avatars/avatar-1.webp" className="rounded float-right" alt="aligment" />
</MDBCol>
</MDBRow>
<MDBRow>
<MDBCol>
<img src="https://mdbootstrap.com/img/Photos/Avatars/avatar-1.webp" className="rounded mx-auto d-block" alt="aligment" />
</MDBCol>
</MDBRow>
Picture
If you are using the <picture>
element to specify multiple <source>
elements
for a specific , make sure to add the .img-* classes to the
<img>
and not to the <picture>
tag.
Images with shadows
import React from "react";
import { MDBContainer, MDBRow, MDBCol } from "mdbreact";
class MasksPage extends React.Component {
render() {
return (
<MDBContainer className="mt-5">
<MDBRow>
<MDBCol lg="4" md="12" className="mb-4">
<img src="https://mdbootstrap.com/img/Others/documentation/img%20(55)-mini.webp" className="img-fluid z-depth-1" alt="" />
</MDBCol>
<MDBCol lg="4" md="6" className="mb-4">
<img src="https://mdbootstrap.com/img/Others/documentation/img%20(55)-mini.webp" className="img-fluid z-depth-1-half"
alt="" />
</MDBCol>
<MDBCol lg="4" md="6" className="mb-4">
<img src="https://mdbootstrap.com/img/Others/documentation/img%20(55)-mini.webp" className="img-fluid z-depth-2" alt="" />
</MDBCol>
</MDBRow>
<MDBRow>
<MDBCol lg="4" md="12" className="mb-4">
<img src="https://mdbootstrap.com/img/Others/documentation/img%20(55)-mini.webp" className="img-fluid z-depth-3" alt="" />
</MDBCol>
<MDBCol lg="4" md="6" className="mb-4">
<img src="https://mdbootstrap.com/img/Others/documentation/img%20(55)-mini.webp" className="img-fluid z-depth-4" alt="" />
</MDBCol>
<MDBCol lg="4" md="6" className="mb-4">
<img src="https://mdbootstrap.com/img/Others/documentation/img%20(55)-mini.webp" className="img-fluid z-depth-5" alt="" />
</MDBCol>
</MDBRow>
</MDBContainer>
);
}
}
export default MasksPage;
Images with overlays
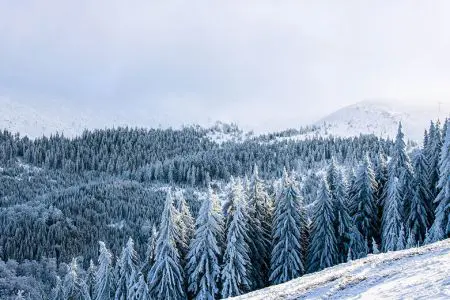
super light overlay
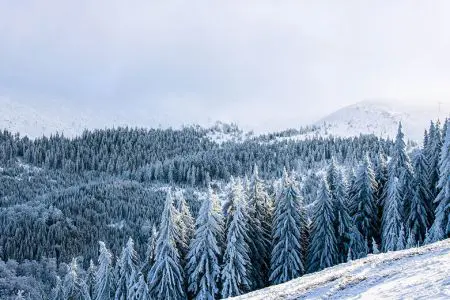
light overlay
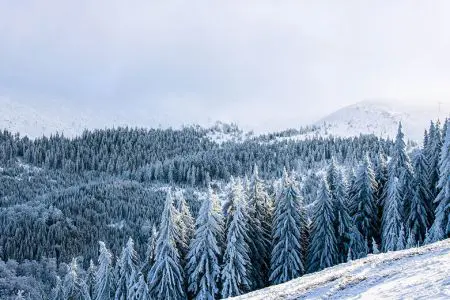
strong overlay
Info notification
Take a look at our masks section to know all the colors possibilities.
import React from "react";
import { MDBMask, MDBView, MDBContainer, MDBRow, MDBCol } from "mdbreact";
class MasksPage extends React.Component {
render() {
return (
<MDBContainer className="mt-5">
<MDBRow className="mt-4">
<MDBCol md="4">
<MDBView>
<img
src="https://mdbootstrap.com/img/Others/documentation/forest-sm-mini.webp"
className="img-fluid"
alt=""
/>
<MDBMask className="flex-center" overlay="teal-slight">
<p className="white-text">super light overlay</p>
</MDBMask>
</MDBView>
</MDBCol>
<MDBCol md="4">
<MDBView>
<img
src="https://mdbootstrap.com/img/Others/documentation/forest-sm-mini.webp"
className="img-fluid"
alt=""
/>
<MDBMask className="flex-center" overlay="teal-light">
<p className="white-text">light overlay</p>
</MDBMask>
</MDBView>
</MDBCol>
<MDBCol md="4">
<MDBView waves>
<img
src="https://mdbootstrap.com/img/Others/documentation/forest-sm-mini.webp"
className="img-fluid"
alt=""
/>
<MDBMask className="flex-center" overlay="teal-strong">
<p className="white-text">strong overlay</p>
</MDBMask>
</MDBView>
</MDBCol>
</MDBRow>
</MDBContainer>
);
}
}
export default MasksPage;
Images with patterns
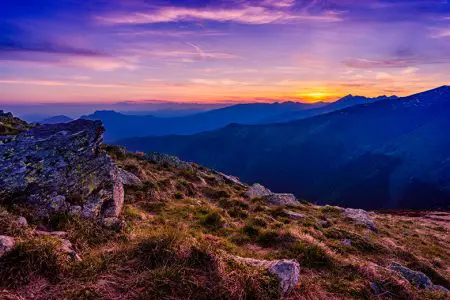
.pattern-1
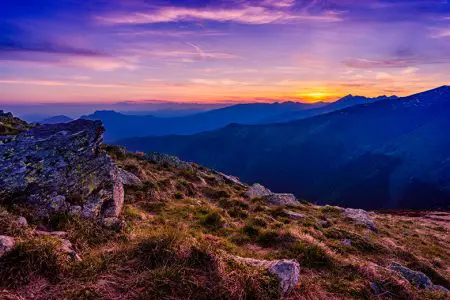
.pattern-2
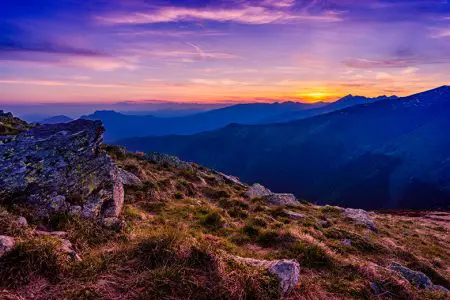
.pattern-3
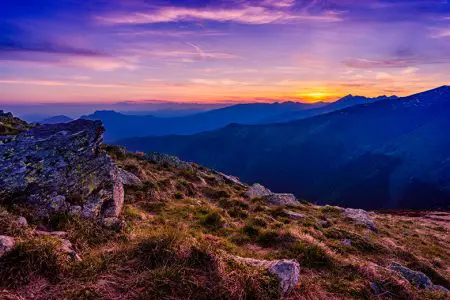
.pattern-4
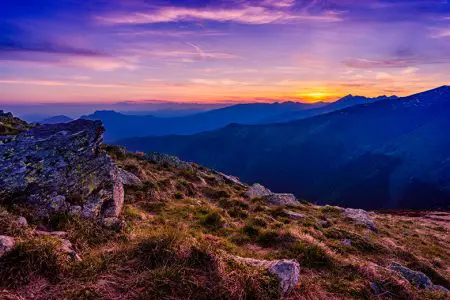
.pattern-5
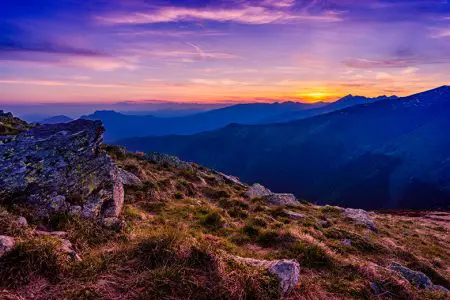
.pattern-6
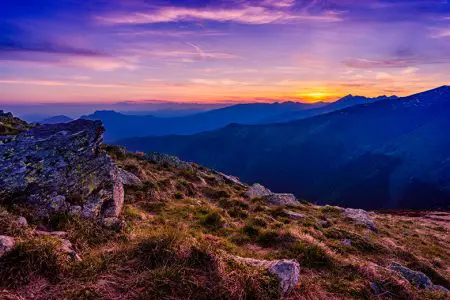
.pattern-7
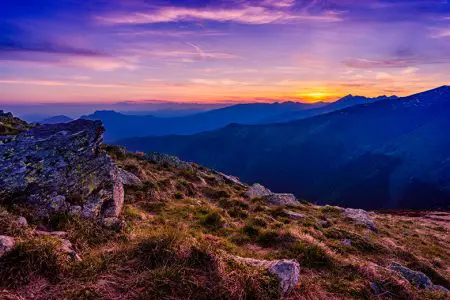
.pattern-8
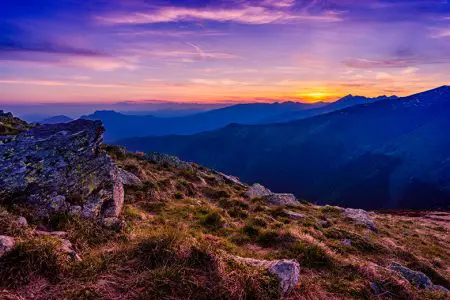
.pattern-9
import React from "react";
import { MDBMask, MDBView, MDBContainer, MDBRow, MDBCol } from "mdbreact";
class MasksPage extends React.Component {
render() {
return (
<MDBContainer className="mt-5">
<MDBRow>
<MDBCol md="4">
<MDBView>
<img
src="https://mdbootstrap.com/img/Photos/Others/nature-sm.webp"
className="img-fluid"
alt=""
/>
<MDBMask pattern={1} className="flex-center">
<p className="white-text">patern 1</p>
</MDBMask>
</MDBView>
</MDBCol>
<MDBCol md="4">
<MDBView>
<img
src="https://mdbootstrap.com/img/Photos/Others/nature-sm.webp"
className="img-fluid"
alt=""
/>
<MDBMask pattern={2} className="flex-center">
<p className="white-text">patern 2</p>
</MDBMask>
</MDBView>
</MDBCol>
<MDBCol md="4">
<MDBView waves>
<img
src="https://mdbootstrap.com/img/Photos/Others/nature-sm.webp"
className="img-fluid"
alt=""
/>
<MDBMask pattern={3} className="flex-center">
<p className="white-text">patern 3</p>
</MDBMask>
</MDBView>
</MDBCol>
</MDBRow>
<MDBRow className="mt-4">
<MDBCol md="4">
<MDBView>
<img
src="https://mdbootstrap.com/img/Photos/Others/nature-sm.webp"
className="img-fluid"
alt=""
/>
<MDBMask className="flex-center" pattern={4}>
<p className="white-text">patern 4</p>
</MDBMask>
</MDBView>
</MDBCol>
<MDBCol md="4">
<MDBView>
<img
src="https://mdbootstrap.com/img/Photos/Others/nature-sm.webp"
className="img-fluid"
alt=""
/>
<MDBMask className="flex-center" pattern={5}>
<p className="white-text">patern 5</p>
</MDBMask>
</MDBView>
</MDBCol>
<MDBCol md="4">
<MDBView waves>
<img
src="https://mdbootstrap.com/img/Photos/Others/nature-sm.webp"
className="img-fluid"
alt=""
/>
<MDBMask className="flex-center" pattern={6}>
<p className="white-text">patern 6</p>
</MDBMask>
</MDBView>
</MDBCol>
</MDBRow>
<MDBRow className="mt-4">
<MDBCol md="4">
<MDBView>
<img
src="https://mdbootstrap.com/img/Photos/Others/nature-sm.webp"
className="img-fluid"
alt=""
/>
<MDBMask className="flex-center" pattern={7}>
<p className="white-text">patern 7</p>
</MDBMask>
</MDBView>
</MDBCol>
<MDBCol md="4">
<MDBView>
<img
src="https://mdbootstrap.com/img/Photos/Others/nature-sm.webp"
className="img-fluid"
alt=""
/>
<MDBMask className="flex-center" pattern={8}>
<p className="white-text">patern 8</p>
</MDBMask>
</MDBView>
</MDBCol>
<MDBCol md="4">
<MDBView waves>
<img
src="https://mdbootstrap.com/img/Photos/Others/nature-sm.webp"
className="img-fluid"
alt=""
/>
<MDBMask className="flex-center" pattern={9}>
<p className="white-text">patern 9</p>
</MDBMask>
</MDBView>
</MDBCol>
</MDBRow>
</MDBContainer>
);
}
}
export default MasksPage;
Images with hover effects
Shadow effect
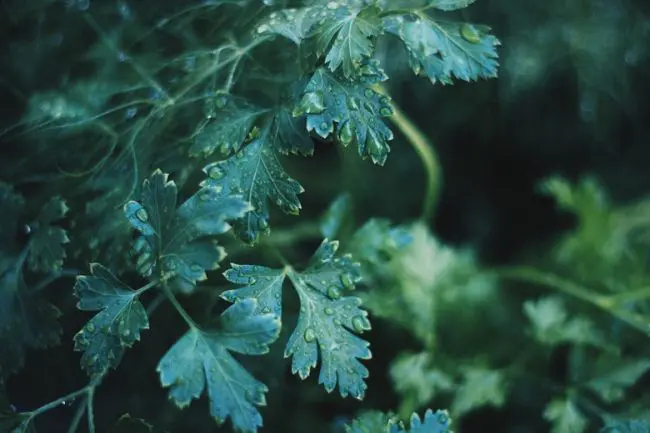
import React from "react";
import { MDBMask, MDBView, MDBContainer, MDBRow, MDBCol } from "mdbreact";
class HoverPage extends React.Component {
render() {
return (
<MDBContainer className="mt-5">
<MDBRow>
<MDBCol md="4">
<MDBView hover>
<img
src="https://mdbootstrap.com/img/Others/documentation/forest-sm-mini.webp"
className="img-fluid"
alt=""
/>
<MDBMask className="flex-center" overlay="red-strong">
<p className="white-text">Strong overlay</p>
</MDBMask>
</MDBView>
</MDBCol>
<MDBCol md="4">
<MDBView hover>
<img
src="https://mdbootstrap.com/img/Others/documentation/forest-sm-mini.webp"
className="img-fluid"
alt=""
/>
<MDBMask className="flex-center" overlay="red-light">
<p className="white-text">Light overlay</p>
</MDBMask>
</MDBView>
</MDBCol>
<MDBCol md="4">
<MDBView hover>
<img
src="https://mdbootstrap.com/img/Others/documentation/forest-sm-mini.webp"
className="img-fluid"
alt=""
/>
<MDBMask className="flex-center" overlay="red-slight">
<p className="white-text">Super light overlay</p>
</MDBMask>
</MDBView>
</MDBCol>
</MDBRow>
<MDBRow className="mt-4">
<MDBCol md="6">
<MDBView hover zoom>
<img
src="https://mdbootstrap.com/img/Others/documentation/img%20(131)-mini.webp"
className="img-fluid"
alt=""
/>
<MDBMask className="flex-center">
<p className="white-text">Zoom effect</p>
</MDBMask>
</MDBView>
</MDBCol>
<MDBCol md="6">
<h5 className="text-center">Shadow effect</h5>
<img
src="https://mdbootstrap.com/img/Others/documentation/4.webp"
className="img-fluid rounded-circle hoverable"
alt=""
/>
</MDBCol>
</MDBRow>
</MDBContainer>
);
}
}
export default HoverPage;
Images with waves effects
Click on the images to see the effect
import React, { Component } from "react";
import {
MDBRow,
MDBCol,
MDBContainer,
MDBView
} from "mdbreact";
class CarouselPage extends Component {
render() {
return (
<MDBContainer>
<MDBRow>
<MDBCol lg="6">
<MDBView waves>
<img src="https://mdbootstrap.com/img/Others/documentation/img%20(1)2-mini.webp" className="img-fluid" alt="" />
</MDBView>
</MDBCol>
<MDBCol lg="6">
<MDBView waves>
<img src="https://mdbootstrap.com/img/Others/documentation/img%20(7)-mini.webp" className="img-fluid" alt="" />
</MDBView>
</MDBCol>
</MDBRow>
</MDBContainer>
);
}
}
export default CarouselPage;
Images within lightbox MDB Pro component
import React from "react";
import { MDBContainer, MDBRow, MDBCol } from "mdbreact";
import Lightbox from "react-image-lightbox";
import "./index.css";
const images = [
"https://mdbootstrap.com/img/Others/documentation/img%20(145)-mini.webp",
"https://mdbootstrap.com/img/Others/documentation/img%20(150)-mini.webp",
"https://mdbootstrap.com/img/Others/documentation/img%20(152)-mini.webp",
"https://mdbootstrap.com/img/Others/documentation/img%20(42)-mini.webp",
"https://mdbootstrap.com/img/Others/documentation/img%20(151)-mini.webp",
"https://mdbootstrap.com/img/Others/documentation/img%20(40)-mini.webp",
"https://mdbootstrap.com/img/Others/documentation/img%20(148)-mini.webp",
"https://mdbootstrap.com/img/Others/documentation/img%20(147)-mini.webp",
"https://mdbootstrap.com/img/Others/documentation/img%20(149)-mini.webp"
];
const smallImages = [
"https://mdbootstrap.com/img/Others/documentation/img%20(145)-mini.webp",
"https://mdbootstrap.com/img/Others/documentation/img%20(150)-mini.webp",
"https://mdbootstrap.com/img/Others/documentation/img%20(152)-mini.webp",
"https://mdbootstrap.com/img/Others/documentation/img%20(42)-mini.webp",
"https://mdbootstrap.com/img/Others/documentation/img%20(151)-mini.webp",
"https://mdbootstrap.com/img/Others/documentation/img%20(40)-mini.webp",
"https://mdbootstrap.com/img/Others/documentation/img%20(148)-mini.webp",
"https://mdbootstrap.com/img/Others/documentation/img%20(147)-mini.webp",
"https://mdbootstrap.com/img/Others/documentation/img%20(149)-mini.webp"
];
class LightboxPage extends React.Component {
constructor(props) {
super(props);
this.state = {
photoIndex: 0,
isOpen: false
};
}
render() {
const { photoIndex, isOpen } = this.state;
return (
<MDBContainer>
<div className="mdb-lightbox no-margin">
<MDBRow>
<MDBCol md="4">
<figure>
<img
src={smallImages[0]}
alt="Gallery"
className="img-fluid"
onClick={() =>
this.setState({ photoIndex: 0, isOpen: true })
}
/>
</figure>
</MDBCol>
<MDBCol md="4">
<figure>
<img
src={smallImages[1]}
alt="Gallery"
className="img-fluid"
onClick={() =>
this.setState({ photoIndex: 1, isOpen: true })
}
/>
</figure>
</MDBCol>
<MDBCol md="4">
<figure>
<img
src={smallImages[2]}
alt="Gallery"
className="img-fluid"
onClick={() =>
this.setState({ photoIndex: 2, isOpen: true })
}
/>
</figure>
</MDBCol>
<MDBCol md="4">
<figure>
<img
src={smallImages[3]}
alt="Gallery"
className="img-fluid"
onClick={() =>
this.setState({ photoIndex: 3, isOpen: true })
}
/>
</figure>
</MDBCol>
<MDBCol md="4">
<figure>
<img
src={smallImages[4]}
alt="Gallery"
className="img-fluid"
onClick={() =>
this.setState({ photoIndex: 4, isOpen: true })
}
/>
</figure>
</MDBCol>
<MDBCol md="4">
<figure>
<img
src={smallImages[5]}
alt="Gallery"
className="img-fluid"
onClick={() =>
this.setState({ photoIndex: 5, isOpen: true })
}
/>
</figure>
</MDBCol>
<MDBCol md="4">
<figure>
<img
src={smallImages[6]}
alt="Gallery"
className="img-fluid"
onClick={() =>
this.setState({ photoIndex: 6, isOpen: true })
}
/>
</figure>
</MDBCol>
<MDBCol md="4">
<figure>
<img
src={smallImages[7]}
alt="Gallery"
className="img-fluid"
onClick={() =>
this.setState({ photoIndex: 7, isOpen: true })
}
/>
</figure>
</MDBCol>
<MDBCol md="4">
<figure>
<img
src={smallImages[8]}
alt="Gallery"
className="img-fluid"
onClick={() =>
this.setState({ photoIndex: 8, isOpen: true })
}
/>
</figure>
</MDBCol>
</MDBRow>
</div>
{isOpen && (
<Lightbox
mainSrc={images[photoIndex]}
nextSrc={images[(photoIndex + 1) % images.length]}
prevSrc={images[(photoIndex + images.length - 1) % images.length]}
imageTitle={photoIndex + 1 + "/" + images.length}
onCloseRequest={() => this.setState({ isOpen: false })}
onMovePrevRequest={() =>
this.setState({
photoIndex: (photoIndex + images.length - 1) % images.length
})
}
onMoveNextRequest={() =>
this.setState({
photoIndex: (photoIndex + 1) % images.length
})
}
/>
)}
</MDBContainer>
);
}
}
export default LightboxPage;
@-webkit-keyframes closeWindow {
0% {
opacity: 1;
}
100% {
opacity: 0;
}
}
@keyframes closeWindow {
0% {
opacity: 1;
}
100% {
opacity: 0;
}
}
.ril__outer {
background-color: rgba(0, 0, 0, 0.85);
outline: none;
top: 0;
left: 0;
right: 0;
bottom: 0;
z-index: 1000;
width: 100%;
height: 100%;
-ms-content-zooming: none;
-ms-user-select: none;
-ms-touch-select: none;
-ms-touch-action: none;
touch-action: none;
}
.ril__outerClosing {
opacity: 0;
}
.ril__inner {
position: absolute;
top: 0;
left: 0;
right: 0;
bottom: 0;
}
.ril__image,
.ril__imagePrev,
.ril__imageNext {
position: absolute;
top: 0;
right: 0;
bottom: 0;
left: 0;
margin: auto;
max-width: none;
-ms-content-zooming: none;
-ms-user-select: none;
-ms-touch-select: none;
-ms-touch-action: none;
touch-action: none;
}
.ril__imageDiscourager {
background-repeat: no-repeat;
background-position: center;
background-size: contain;
}
.ril__navButtons {
border: none;
position: absolute;
top: 0;
bottom: 0;
width: 20px;
height: 34px;
padding: 40px 30px;
margin: auto;
cursor: pointer;
opacity: 0.7;
}
.ril__navButtons:hover {
opacity: 1;
}
.ril__navButtons:active {
opacity: 0.7;
}
.ril__navButtonPrev {
left: 0;
background: rgba(0, 0, 0, 0.2)
url('data:image/svg+xml;base64,PHN2ZyB4bWxucz0iaHR0cDovL3d3dy53My5vcmcvMjAwMC9zdmciIHZlcnNpb249IjEuMSIgd2lkdGg9IjIwIiBoZWlnaHQ9IjM0Ij48cGF0aCBkPSJtIDE5LDMgLTIsLTIgLTE2LDE2IDE2LDE2IDEsLTEgLTE1LC0xNSAxNSwtMTUgeiIgZmlsbD0iI0ZGRiIvPjwvc3ZnPg==')
no-repeat center;
}
.ril__navButtonNext {
right: 0;
background: rgba(0, 0, 0, 0.2)
url('data:image/svg+xml;base64,PHN2ZyB4bWxucz0iaHR0cDovL3d3dy53My5vcmcvMjAwMC9zdmciIHZlcnNpb249IjEuMSIgd2lkdGg9IjIwIiBoZWlnaHQ9IjM0Ij48cGF0aCBkPSJtIDEsMyAyLC0yIDE2LDE2IC0xNiwxNiAtMSwtMSAxNSwtMTUgLTE1LC0xNSB6IiBmaWxsPSIjRkZGIi8+PC9zdmc+')
no-repeat center;
}
.ril__downloadBlocker {
position: absolute;
top: 0;
left: 0;
right: 0;
bottom: 0;
background-image: url('data:image/gif;base64,R0lGODlhAQABAIAAAAAAAP///yH5BAEAAAAALAAAAAABAAEAAAIBRAA7');
background-size: cover;
}
.ril__caption,
.ril__toolbar {
background-color: rgba(0, 0, 0, 0.5);
position: absolute;
left: 0;
right: 0;
display: -webkit-box;
display: -ms-flexbox;
display: flex;
-webkit-box-pack: justify;
-ms-flex-pack: justify;
justify-content: space-between;
}
.ril__caption {
bottom: 0;
max-height: 150px;
overflow: auto;
}
.ril__captionContent {
padding: 10px 20px;
color: #fff;
}
.ril__toolbar {
top: 0;
height: 50px;
}
.ril__toolbarSide {
height: 50px;
margin: 0;
}
.ril__toolbarLeftSide {
padding-left: 20px;
padding-right: 0;
-webkit-box-flex: 0;
-ms-flex: 0 1 auto;
flex: 0 1 auto;
overflow: hidden;
text-overflow: ellipsis;
}
.ril__toolbarRightSide {
padding-left: 0;
padding-right: 20px;
-webkit-box-flex: 0;
-ms-flex: 0 0 auto;
flex: 0 0 auto;
}
.ril__toolbarItem {
display: inline-block;
line-height: 50px;
padding: 0;
color: #fff;
font-size: 120%;
max-width: 100%;
overflow: hidden;
text-overflow: ellipsis;
white-space: nowrap;
}
.ril__toolbarItemChild {
vertical-align: middle;
}
.ril__builtinButton {
width: 40px;
height: 35px;
cursor: pointer;
border: none;
opacity: 0.7;
}
.ril__builtinButton:hover {
opacity: 1;
}
.ril__builtinButton:active {
outline: none;
}
.ril__builtinButtonDisabled {
cursor: default;
opacity: 0.5;
}
.ril__builtinButtonDisabled:hover {
opacity: 0.5;
}
.ril__closeButton {
background: url('data:image/svg+xml;base64,PHN2ZyB4bWxucz0iaHR0cDovL3d3dy53My5vcmcvMjAwMC9zdmciIHZlcnNpb249IjEuMSIgd2lkdGg9IjIwIiBoZWlnaHQ9IjIwIj48cGF0aCBkPSJtIDEsMyAxLjI1LC0xLjI1IDcuNSw3LjUgNy41LC03LjUgMS4yNSwxLjI1IC03LjUsNy41IDcuNSw3LjUgLTEuMjUsMS4yNSAtNy41LC03LjUgLTcuNSw3LjUgLTEuMjUsLTEuMjUgNy41LC03LjUgLTcuNSwtNy41IHoiIGZpbGw9IiNGRkYiLz48L3N2Zz4=')
no-repeat center;
}
.ril__zoomInButton {
background: url('data:image/svg+xml;base64,PHN2ZyB4bWxucz0iaHR0cDovL3d3dy53My5vcmcvMjAwMC9zdmciIHdpZHRoPSIyMCIgaGVpZ2h0PSIyMCI+PGcgc3Ryb2tlPSIjZmZmIiBzdHJva2Utd2lkdGg9IjIiIHN0cm9rZS1saW5lY2FwPSJyb3VuZCI+PHBhdGggZD0iTTEgMTlsNi02Ii8+PHBhdGggZD0iTTkgOGg2Ii8+PHBhdGggZD0iTTEyIDV2NiIvPjwvZz48Y2lyY2xlIGN4PSIxMiIgY3k9IjgiIHI9IjciIGZpbGw9Im5vbmUiIHN0cm9rZT0iI2ZmZiIgc3Ryb2tlLXdpZHRoPSIyIi8+PC9zdmc+')
no-repeat center;
}
.ril__zoomOutButton {
background: url('data:image/svg+xml;base64,PHN2ZyB4bWxucz0iaHR0cDovL3d3dy53My5vcmcvMjAwMC9zdmciIHdpZHRoPSIyMCIgaGVpZ2h0PSIyMCI+PGcgc3Ryb2tlPSIjZmZmIiBzdHJva2Utd2lkdGg9IjIiIHN0cm9rZS1saW5lY2FwPSJyb3VuZCI+PHBhdGggZD0iTTEgMTlsNi02Ii8+PHBhdGggZD0iTTkgOGg2Ii8+PC9nPjxjaXJjbGUgY3g9IjEyIiBjeT0iOCIgcj0iNyIgZmlsbD0ibm9uZSIgc3Ryb2tlPSIjZmZmIiBzdHJva2Utd2lkdGg9IjIiLz48L3N2Zz4=')
no-repeat center;
}
.ril__outerAnimating {
-webkit-animation-name: closeWindow;
animation-name: closeWindow;
}
@-webkit-keyframes pointFade {
0%,
19.999%,
100% {
opacity: 0;
}
20% {
opacity: 1;
}
}
@keyframes pointFade {
0%,
19.999%,
100% {
opacity: 0;
}
20% {
opacity: 1;
}
}
.ril__loadingCircle {
width: 60px;
height: 60px;
position: relative;
}
.ril__loadingCirclePoint {
width: 100%;
height: 100%;
position: absolute;
left: 0;
top: 0;
}
.ril__loadingCirclePoint::before {
content: '';
display: block;
margin: 0 auto;
width: 11%;
height: 30%;
background-color: #fff;
border-radius: 30%;
-webkit-animation: pointFade 800ms infinite ease-in-out both;
animation: pointFade 800ms infinite ease-in-out both;
}
.ril__loadingCirclePoint:nth-of-type(1) {
-webkit-transform: rotate(0deg);
transform: rotate(0deg);
}
.ril__loadingCirclePoint:nth-of-type(7) {
-webkit-transform: rotate(180deg);
transform: rotate(180deg);
}
.ril__loadingCirclePoint:nth-of-type(1)::before,
.ril__loadingCirclePoint:nth-of-type(7)::before {
-webkit-animation-delay: -800ms;
animation-delay: -800ms;
}
.ril__loadingCirclePoint:nth-of-type(2) {
-webkit-transform: rotate(30deg);
transform: rotate(30deg);
}
.ril__loadingCirclePoint:nth-of-type(8) {
-webkit-transform: rotate(210deg);
transform: rotate(210deg);
}
.ril__loadingCirclePoint:nth-of-type(2)::before,
.ril__loadingCirclePoint:nth-of-type(8)::before {
-webkit-animation-delay: -666ms;
animation-delay: -666ms;
}
.ril__loadingCirclePoint:nth-of-type(3) {
-webkit-transform: rotate(60deg);
transform: rotate(60deg);
}
.ril__loadingCirclePoint:nth-of-type(9) {
-webkit-transform: rotate(240deg);
transform: rotate(240deg);
}
.ril__loadingCirclePoint:nth-of-type(3)::before,
.ril__loadingCirclePoint:nth-of-type(9)::before {
-webkit-animation-delay: -533ms;
animation-delay: -533ms;
}
.ril__loadingCirclePoint:nth-of-type(4) {
-webkit-transform: rotate(90deg);
transform: rotate(90deg);
}
.ril__loadingCirclePoint:nth-of-type(10) {
-webkit-transform: rotate(270deg);
transform: rotate(270deg);
}
.ril__loadingCirclePoint:nth-of-type(4)::before,
.ril__loadingCirclePoint:nth-of-type(10)::before {
-webkit-animation-delay: -400ms;
animation-delay: -400ms;
}
.ril__loadingCirclePoint:nth-of-type(5) {
-webkit-transform: rotate(120deg);
transform: rotate(120deg);
}
.ril__loadingCirclePoint:nth-of-type(11) {
-webkit-transform: rotate(300deg);
transform: rotate(300deg);
}
.ril__loadingCirclePoint:nth-of-type(5)::before,
.ril__loadingCirclePoint:nth-of-type(11)::before {
-webkit-animation-delay: -266ms;
animation-delay: -266ms;
}
.ril__loadingCirclePoint:nth-of-type(6) {
-webkit-transform: rotate(150deg);
transform: rotate(150deg);
}
.ril__loadingCirclePoint:nth-of-type(12) {
-webkit-transform: rotate(330deg);
transform: rotate(330deg);
}
.ril__loadingCirclePoint:nth-of-type(6)::before,
.ril__loadingCirclePoint:nth-of-type(12)::before {
-webkit-animation-delay: -133ms;
animation-delay: -133ms;
}
.ril__loadingCirclePoint:nth-of-type(7) {
-webkit-transform: rotate(180deg);
transform: rotate(180deg);
}
.ril__loadingCirclePoint:nth-of-type(13) {
-webkit-transform: rotate(360deg);
transform: rotate(360deg);
}
.ril__loadingCirclePoint:nth-of-type(7)::before,
.ril__loadingCirclePoint:nth-of-type(13)::before {
-webkit-animation-delay: 0ms;
animation-delay: 0ms;
}
.ril__loadingContainer {
position: absolute;
top: 0;
right: 0;
bottom: 0;
left: 0;
}
.ril__imagePrev .ril__loadingContainer,
.ril__imageNext .ril__loadingContainer {
display: none;
}
.ril__errorContainer {
position: absolute;
top: 0;
right: 0;
bottom: 0;
left: 0;
display: -webkit-box;
display: -ms-flexbox;
display: flex;
-webkit-box-align: center;
-ms-flex-align: center;
align-items: center;
-webkit-box-pack: center;
-ms-flex-pack: center;
justify-content: center;
color: #fff;
}
.ril__imagePrev .ril__errorContainer,
.ril__imageNext .ril__errorContainer {
display: none;
}
.ril__loadingContainer__icon {
color: #fff;
position: absolute;
top: 50%;
left: 50%;
-webkit-transform: translateX(-50%) translateY(-50%);
transform: translateX(-50%) translateY(-50%);
}
Images within cards MDB Pro component
Culinary
Cheat day inspirations
Ut enim ad minima veniam, quis nostrum exercitationem ullam corporis suscipit laboriosam, nisi ut aliquid ex ea commodi.
Button
import React, { Component } from 'react';
import { MDBBtn, MDBCard, MDBCardBody, MDBCardImage, MDBCardTitle, MDBCardText, MDBRow, MDBCol } from 'mdbreact';
class CardExample extends Component {
render() {
return (
<MDBRow>
<MDBCol>
<MDBCard wide>
<MDBCardImage cascade className="img-fluid" src="https://mdbootstrap.com/img/Photos/Horizontal/People/6-col/img%20%283%29.webp" />
<MDBCardBody cascade>
<MDBCardTitle>MDBCard title</MDBCardTitle>
<MDBCardText>Some quick example text to build on the card title and make up the bulk of the card's content.</MDBCardText>
<MDBBtn href="#">MDBBtn</MDBBtn>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol>
<MDBCard narrow>
<MDBCardImage cascade className="img-fluid" src="https://mdbootstrap.com/img/Photos/Lightbox/Thumbnail/img%20(147).webp" />
<MDBCardBody cascade>
<MDBCardTitle>MDBCard title</MDBCardTitle>
<MDBCardText>Some quick example text to build on the card title and make up the bulk of the card's content.</MDBCardText>
<MDBBtn href="#">MDBBtn</MDBBtn>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol>
<MDBCard cascade>
<MDBCardImage cascade className="img-fluid" src="https://mdbootstrap.com/img/Photos/Others/men.webp" />
<MDBCardBody cascade>
<MDBCardTitle>MDBCard title</MDBCardTitle>
<MDBCardText>Some quick example text to build on the card title and make up the bulk of the card's content.</MDBCardText>
<MDBBtn href="#">MDBBtn</MDBBtn>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
)
}
}
export default CardExample;
Images within magazine section MDB Pro component
Section title
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit id laborum.
import React from "react";
import { MDBRow, MDBCol, MDBCard, MDBCardBody, MDBMask, MDBIcon, MDBView, MDBBadge } from "mdbreact";
const FeaturesPage = () => {
const newsStyle = {
borderBottom: "1px solid #e0e0e0",
marginBottom: "1.5rem"
};
return (
<MDBCard
className="my-5 px-5 mx-auto"
style={{ fontWeight: 300, maxWidth: "90%" }}
>
<MDBCardBody style={{ paddingTop: 0 }}>
<h2 className="h1-responsive font-weight-bold my-5 text-center">
Section title
</h2>
<p className="dark-grey-text mx-auto mb-5 w-75 text-center">
Duis aute irure dolor in reprehenderit in voluptate velit esse
cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat
cupidatat non proident, sunt in culpa qui officia deserunt mollit id
laborum.
</p>
<MDBRow>
<MDBCol md="12" lg="4" className="mb-lg-0 mb-5">
<MDBView hover rounded className="z-depth-1-half mb-4">
<img
className="img-fluid"
src="https://mdbootstrap.com/img/Photos/Others/images/86.webp"
alt=""
/>
<a href="#!">
<MDBMask overlay="white-slight" className="waves-light" />
</a>
</MDBView>
<MDBRow className="mb-3">
<MDBCol size="12">
<a href="#!">
<MDBBadge color="pink">
<MDBIcon icon="camera" className="pr-2" aria-hidden="true" />
Adventure
</MDBBadge>
</a>
</MDBCol>
</MDBRow>
<div className="d-flex justify-content-between" style={newsStyle}>
<MDBCol size="11" className="text-truncate pl-0 mb-3">
<a href="#!" className="font-weight-bold">
This is title of the news
</a>
</MDBCol>
<a href="#!">
<MDBIcon icon="angle-double-right" />
</a>
</div>
<div className="d-flex justify-content-between" style={newsStyle}>
<MDBCol size="11" className="text-truncate pl-0 mb-3">
<a href="#!">24 Food Swaps That Slash Calories.</a>
</MDBCol>
<a href="#!">
<MDBIcon icon="angle-double-right" />
</a>
</div>
<div className="d-flex justify-content-between" style={newsStyle}>
<MDBCol size="11" className="text-truncate pl-0 mb-3">
<a href="#!">How to Make a Beet Cocktail?</a>
</MDBCol>
<a href="#!">
<MDBIcon icon="angle-double-right" />
</a>
</div>
<div className="d-flex justify-content-between" style={newsStyle}>
<MDBCol size="11" className="text-truncate pl-0 mb-3">
<a href="#!">8 Sneaky Reasons You're Always Hungry.</a>
</MDBCol>
<a href="#!">
<MDBIcon icon="angle-double-right" />
</a>
</div>
<div className="d-flex justify-content-between mb-4">
<MDBCol size="11" className="text-truncate pl-0 mb-3">
<a href="#!">5 Pressure Cooker Recipes You Need to Try.</a>
</MDBCol>
<a href="#!">
<MDBIcon icon="angle-double-right" />
</a>
</div>
</MDBCol>
<MDBCol md="12" lg="4" className="mb-lg-0 mb-5">
<MDBView hover rounded className="z-depth-1-half mb-4">
<img
className="img-fluid"
src="https://mdbootstrap.com/img/Photos/Others/images/31.webp"
alt=""
/>
<a href="#!">
<MDBMask overlay="white-slight" className="waves-light" />
</a>
</MDBView>
<MDBRow className="mb-3">
<MDBCol size="12">
<a href="#!">
<MDBBadge color="deep-orange">
<MDBIcon icon="plane" className="pr-2" aria-hidden="true" />
Travel
</MDBBadge>
</a>
</MDBCol>
</MDBRow>
<div className="d-flex justify-content-between" style={newsStyle}>
<MDBCol size="11" className="text-truncate pl-0 mb-3">
<a href="#!" className="font-weight-bold">
This is title of the news
</a>
</MDBCol>
<a href="#!">
<MDBIcon icon="angle-double-right" />
</a>
</div>
<div className="d-flex justify-content-between" style={newsStyle}>
<MDBCol size="11" className="text-truncate pl-0 mb-3">
<a href="#!">Trends in the blogosphere for 2018.</a>
</MDBCol>
<a href="#!">
<MDBIcon icon="angle-double-right" />
</a>
</div>
<div className="d-flex justify-content-between" style={newsStyle}>
<MDBCol size="11" className="text-truncate pl-0 mb-3">
<a href="#!">Where can you eat the best lunch in Warsaw?</a>
</MDBCol>
<a href="#!">
<MDBIcon icon="angle-double-right" />
</a>
</div>
<div className="d-flex justify-content-between" style={newsStyle}>
<MDBCol size="11" className="text-truncate pl-0 mb-3">
<a href="#!">What camera is worth taking for holidays?</a>
</MDBCol>
<a href="#!">
<MDBIcon icon="angle-double-right" />
</a>
</div>
<div className="d-flex justify-content-between mb-4">
<MDBCol size="11" className="text-truncate pl-0 mb-3">
<a href="#!">Why should you visit Lisbon?</a>
</MDBCol>
<a href="#!">
<MDBIcon icon="angle-double-right" />
</a>
</div>
</MDBCol>
<MDBCol md="12" lg="4" className="mb-lg-0 mb-5">
<MDBView hover rounded className="z-depth-1-half mb-4">
<img
className="img-fluid"
src="https://mdbootstrap.com/img/Photos/Others/images/52.webp"
alt=""
/>
<a href="#!">
<MDBMask overlay="white-slight" className="waves-light" />
</a>
</MDBView>
<MDBRow className="mb-3">
<MDBCol size="12">
<a href="#!">
<MDBBadge color="success">
<MDBIcon icon="leaf" className="pr-2" aria-hidden="true" />
Nature
</MDBBadge>
</a>
</MDBCol>
</MDBRow>
<div className="d-flex justify-content-between" style={newsStyle}>
<MDBCol size="11" className="text-truncate pl-0 mb-3">
<a href="#!" className="font-weight-bold">
This is title of the news
</a>
</MDBCol>
<a href="#!">
<MDBIcon icon="angle-double-right" />
</a>
</div>
<div className="d-flex justify-content-between" style={newsStyle}>
<MDBCol size="11" className="text-truncate pl-0 mb-3">
<a href="#!">
How to recognize the footsteps of wild animals?
</a>
</MDBCol>
<a href="#!">
<MDBIcon icon="angle-double-right" />
</a>
</div>
<div className="d-flex justify-content-between" style={newsStyle}>
<MDBCol size="11" className="text-truncate pl-0 mb-3">
<a href="#!">National Parks in Poland.</a>
</MDBCol>
<a href="#!">
<MDBIcon icon="angle-double-right" />
</a>
</div>
<div className="d-flex justify-content-between" style={newsStyle}>
<MDBCol size="11" className="text-truncate pl-0 mb-3">
<a href="#!">Traveling without littering the planet.</a>
</MDBCol>
<a href="#!">
<MDBIcon icon="angle-double-right" />
</a>
</div>
<div className="d-flex justify-content-between mb-4">
<MDBCol size="11" className="text-truncate pl-0 mb-3">
<a href="#!">How to protect rainforests?</a>
</MDBCol>
<a href="#!">
<MDBIcon icon="angle-double-right" />
</a>
</div>
</MDBCol>
</MDBRow>
</MDBCardBody>
</MDBCard>
);
}
export default FeaturesPage;
Images as the avatars within testimonials MDB Pro component
Testimonials
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Fugit, error amet numquam iure provident voluptate esse quasi, veritatis totam voluptas nostrum quisquam eum porro a pariatur accusamus veniam.
-mini.webp)
John Doe
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Eos, adipisci.
-mini.webp)
Anna Aston
Neque cupiditate assumenda in maiores repudiandae mollitia architecto.
-mini.webp)
Maria Kate
Delectus impedit saepe officiis ab aliquam repellat, rem totam unde ducimus.
import React from "react";
import { MDBContainer, MDBRow, MDBCol, MDBCard, MDBCardUp, MDBAvatar, MDBCardBody, MDBIcon } from "mdbreact";
const TestimonialsPage = () => {
return (
<MDBContainer>
<section className="text-center my-5">
<h2 className="h1-responsive font-weight-bold my-5">
Testimonials
</h2>
<p className="dark-grey-text w-responsive mx-auto mb-5">
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Fugit,
error amet numquam iure provident voluptate esse quasi, veritatis
totam voluptas nostrum quisquam eum porro a pariatur veniam.
</p>
<MDBRow>
<MDBCol lg="4" md="12" className="mb-lg-0 mb-4">
<MDBCard testimonial>
<MDBCardUp color="info" />
<MDBAvatar className="mx-auto white">
<img
src="https://mdbootstrap.com/img/Photos/Avatars/img%20(9).webp"
alt=""
className="rounded-circle img-fluid"
/>
</MDBAvatar>
<MDBCardBody>
<h4 className="font-weight-bold mb-4">John Doe</h4>
<hr />
<p className="dark-grey-text mt-4">
<MDBIcon icon="quote-left" className="pr-2" />
Lorem ipsum dolor sit amet eos adipisci, consectetur
adipisicing elit.
</p>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol lg="4" md="6" className="mb-lg-0 mb-4">
<MDBCard testimonial>
<MDBCardUp gradient="blue" />
<MDBAvatar className="mx-auto white">
<img
src="https://mdbootstrap.com/img/Photos/Avatars/img%20(20).webp"
alt=""
className="rounded-circle img-fluid"
/>
</MDBAvatar>
<MDBCardBody>
<h4 className="font-weight-bold mb-4">Anna Aston</h4>
<hr />
<p className="dark-grey-text mt-4">
<i className="fa fa-quote-left pr-2" />
Neque cupiditate assumenda in maiores repudiandae mollitia
architecto.
</p>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol lg="4" md="6" className="mb-lg-0 mb-4">
<MDBCard testimonial>
<MDBCardUp className="indigo" />
<MDBAvatar className="mx-auto white">
<img
src="https://mdbootstrap.com/img/Photos/Avatars/img%20(10).webp"
alt=""
className="rounded-circle img-fluid"
/>
</MDBAvatar>
<MDBCardBody>
<h4 className="font-weight-bold mb-4">Maria Kate</h4>
<hr />
<p className="dark-grey-text mt-4">
<i className="fa fa-quote-left pr-2" />
Delectus impedit saepe officiis ab aliquam repellat rem unde
ducimus.
</p>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
</section>
</MDBContainer>
);
}
export default TestimonialsPage;
Images within grid
import React, { Component } from "react";
import {
MDBRow,
MDBCol,
MDBContainer
} from "mdbreact";
class CarouselPage extends Component {
render() {
return (
<MDBContainer>
<MDBRow>
<MDBCol md="12" className="mb-3">
<img src="https://mdbootstrap.com/img/Photos/Slides/img%20(137).webp" className="img-fluid z-depth-1" alt="" />
</MDBCol>
</MDBRow>
<MDBRow>
<MDBCol lg="4" md="12" className="mb-3">
<img src="https://mdbootstrap.com/img/Others/documentation/img(115)-mini.webp" className="img-fluid z-depth-1" alt="" />
</MDBCol>
<MDBCol lg="4" md="6" className="mb-3">
<img src="https://mdbootstrap.com/img/Others/documentation/img(116)-mini.webp" className="img-fluid z-depth-1" alt="" />
</MDBCol>
<MDBCol lg="4" md="6" className="mb-3">
<img src="https://mdbootstrap.com/img/Others/documentation/img(117)-mini.webp" className="img-fluid z-depth-1" alt="" />
</MDBCol>
</MDBRow>
<MDBRow>
<MDBCol md="6" className="mb-3">
<img src="https://mdbootstrap.com/img/Others/documentation/img(118)-mini.webp" className="img-fluid z-depth-1" alt="" />
</MDBCol>
<MDBCol md="6" className="mb-3">
<img src="https://mdbootstrap.com/img/Others/documentation/img(129)-mini.webp" className="img-fluid z-depth-1" alt="" / >
</MDBCol>
</MDBRow>
</MDBContainer>
);
}
}
export default CarouselPage;
Shapes of images
import React, { Component } from "react";
import {
MDBRow,
MDBCol,
MDBContainer
} from "mdbreact";
class CarouselPage extends Component {
render() {
return (
<MDBContainer>
<MDBRow>
<MDBCol xl="4" md="4" className="mb-3">
<img src="https://mdbootstrap.com/img/Others/documentation/img(119)-mini.webp" className="img-fluid z-depth-1" alt="" />
</MDBCol>
<MDBCol xl="5" md="4" className="mb-3 text-center">
<img src="https://mdbootstrap.com/img/Photos/Avatars/img(31).webp" className="img-fluid z-depth-1 rounded-circle" alt="" />
</MDBCol>
<MDBCol xl="3" md="4" className="mb-3 text-md-right text-center">
<img src="https://mdbootstrap.com/img/Photos/Avatars/img(30).webp" className="img-fluid z-depth-1" alt="" />
</MDBCol>
</MDBRow>
</MDBContainer>
);
}
}
export default CarouselPage;
Images within carousel
import React, { Component } from "react";
import {
MDBCarousel,
MDBCarouselInner,
MDBCarouselItem,
MDBView,
MDBMask,
MDBContainer
} from "mdbreact";
class CarouselPage extends Component {
render() {
return (
<MDBContainer>
<MDBCarousel
activeItem={1}
length={3}
showControls={true}
showIndicators={true}
className="z-depth-1"
>
<MDBCarouselInner>
<MDBCarouselItem itemId="1">
<MDBView>
<img
className="d-block w-100"
src="https://mdbootstrap.com/img/Others/documentation/img%20(136)-mini.webp"
alt="First slide"
/>
<MDBMask overlay="black-light" />
</MDBView>
</MDBCarouselItem>
<MDBCarouselItem itemId="2">
<MDBView>
<img
className="d-block w-100"
src="https://mdbootstrap.com/img/Others/documentation/img%20(137)-mini.webp"
alt="Second slide"
/>
<MDBMask overlay="black-strong" />
</MDBView>
</MDBCarouselItem>
<MDBCarouselItem itemId="3">
<MDBView>
<img
className="d-block w-100"
src="https://mdbootstrap.com/img/Others/documentation/img%20(141)-mini.webp"
alt="Third slide"
/>
<MDBMask overlay="black-slight" />
</MDBView>
</MDBCarouselItem>
</MDBCarouselInner>
</MDBCarousel>
</MDBContainer>
);
}
}
export default CarouselPage;