React Animations
React Animations - Bootstrap 4 & Material Design
React Bootstrap animations are illusions of motions for web elements. +70 animations generated by CSS only, work properly on every browser.
Note: This documentation is for an older version of Bootstrap (v.4). A
newer version is available for Bootstrap 5. We recommend migrating to the latest version of our product - Material Design for
Bootstrap 5.
Go to docs v.5
Basic usage
Using our animation is simple. Wrap the content you want to animate into the Animation
component and specify its type. Check out the API section for more advanced options!
import React from "react";
import { MDBAnimation } from "mdbreact";
const AnimationPage = () => {
return (
<MDBAnimation type="bounce" infinite>
<img className="img-fluid" alt="" src="https://mdbootstrap.com/img/logo/mdb-transparent-250px.webp" />
</MDBAnimation>
);
};
export default AnimationPage;
React Animations - API
In this section you will find advanced information about the Animation component. You will find out which modules are required, what are the possibilities of configuring the component, and what events and methods you can use in working with it.
Animation import statement
In order to use Animation component make sure you have imported proper module first.
import { MDBAnimation } from "mdbreact";
API Reference: Animation Properties
The table below shows the configuration options of the MDBAnimation component.
Name | Type | Default | Description | Example |
---|---|---|---|---|
className |
String |
|
Adds custom classes | <MDBAutocomplete className="myClass" /> |
type |
String |
|
The props defines the kind of animation desired | <MDBAnimation type="bounce" /> |
count |
Number | 1 |
The number of iterations the animation should have | <MDBAnimation count={4} /> |
duration |
String | 1s |
Defines the speed of animation, takes a string consisting of a number and a unit indicator (s /ms ) |
<MDBAnimation duration="5s" /> |
delay |
String | 0 | Sets the amount of time that should pass before the initial iteration of animation, takes a
string consisting of a number and a unit indicator (s /ms ) |
<MDBAnimation delay="5s" /> |
infinite |
Bolean | false |
Makes an animation go for eternity. Note: it overwrites the count prop |
<MDBAnimation infinite /> |
reveal |
Bolean | false |
Setting this option makes the component postpone the animation untill the viewport reaches it's relative position | <MDBAnimation reveal /> |
API Reference: Animation Methods
We can fire events on arbitrary stages of animation. The props below take in functions that resolve whenever the related stage is reached.
Name | Parameters | Description | Example |
---|---|---|---|
onAnimationStart |
Fires once an animation starts | <MDBAnimation onAnimationStart={this.handleStart} > |
|
onAnimationIteration |
Function gets executed every time an animation happens. To have things happening only on particular
iterations (say, every time for the first five times and then every fith one), it is enough to create a
variable being incremented within a function and define a simple if statement to see, whether
the ongoing iteration number fullfills the requirement (like if (count < 6 || count % 5 == 0){yourFunction} ) |
<MDBAnimation onAnimationIteration={this.yourFunction} > |
|
onAnimationEnd |
Function shall take place after the final iteration of an animation | <MDBAnimation onAnimationStart={this.onAnimationEnd} > |
Available animations
Using the Animation
component means having over seventy ready-to-use animation
types. They are inputted as strings into the type
prop.
Full list of animations:
flash
bounce
pulse
rubberBand
shake
headShake
swing
tada
wobble
jello
bounceIn
bounceInDown
bounceInLeft
bounceInRight
bounceInUp
bounceOut
bounceOutDown
bounceOutLeft
bounceOutRight
bounceOutUp
fadeIn
fadeInDown
fadeInDownBig
fadeInLeft
fadeInLeftBig
fadeInRight
fadeInRightBig
fadeInUp
fadeInUpBig
fadeOut
fadeOutDown
fadeOutDownBig
fadeOutLeft
fadeOutLeftBig
fadeOutRight
fadeOutRightBig
fadeOutUp
fadeOutUpBig
flipInX
flipInY
flipOutX
flipOutY
lightSpeedIn
lightSpeedOut
rotateIn
rotateInDownLeft
rotateInDownRight
rotateInUpLeft
rotateInUpRight
rotateOut
rotateOutDownLeft
rotateOutDownRight
rotateOutUpLeft
rotateOutUpRight
hinge
rollIn
rollOut
zoomIn
zoomInDown
zoomInLeft
zoomInRight
zoomInUp
zoomOut
zoomOutDown
zoomOutLeft
zoomOutRight
zoomOutUp
slideInDown
slideInLeft
slideInRight
slideInUp
slideOutDown
slideOutLeft
slideOutRight
slideOutUp
Animation creator
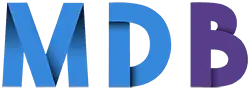
Reveal animations when scrolling
Thanks to MDB you can easily launch an animation on page scroll. It is just as easy as
adding the reveal
prop to the Animation
component!
import React from "react";
import { MDBAnimation } from "mdbreact";
const AnimationPage = () => {
return (
<>
<MDBAnimation reveal type="bounceInUp">
<img alt="A view on mountains." className="img-fluid" src="https://mdbootstrap.com/img/Photos/Horizontal/Nature/4-col/img%20(31).webp" />
</MDBAnimation>
<MDBAnimation reveal type="tada">
<img alt="Cottage on a lake surrounded by mountains." className="img-fluid" src="https://mdbootstrap.com/img/Photos/Horizontal/Nature/4-col/img%20(32).webp" />
</MDBAnimation>
<MDBAnimation reveal type="fadeInLeft">
<img alt="A boat floating on an ocean" className="img-fluid" src="https://mdbootstrap.com/img/Photos/Horizontal/Nature/4-col/img%20(73).webp" />
</MDBAnimation>
<MDBAnimation reveal type="fadeInRight">
<img alt="View on mountains from mountain top." className="img-fluid" src="https://mdbootstrap.com/img/Photos/Horizontal/Nature/4-col/img%20(34).webp" />
</MDBAnimation>
<MDBAnimation reveal type="fadeInRight">
<img alt="Rocky shore in the morning." className="img-fluid" src="https://mdbootstrap.com/img/Photos/Horizontal/Nature/4-col/img%20(14).webp" />
</MDBAnimation>
<MDBAnimation reveal type="fadeInUp">
<img alt="Rocky shore in the morning." className="img-fluid" src="https://mdbootstrap.com/img/Photos/Horizontal/Nature/4-col/img%20(35).webp" />
</MDBAnimation>
</>
);
};
export default AnimationPage;