React Masks
React Masks - Bootstrap 4 & Material Design
Note: This documentation is for an older version of Bootstrap (v.4). A
newer version is available for Bootstrap 5. We recommend migrating to the latest version of our product - Material Design for
Bootstrap 5.
Go to docs v.5
React Bootstrap masks alter the visibility of an element by either partially or fully hiding it. Masks are used to make content more visible in the picture.
Pattern
.webp)
pattern = 8
import React from "react";
import { MDBMask, MDBView, MDBContainer } from "mdbreact";
const HoverPage = () => {
return (
<MDBContainer className="mt-5">
<MDBView>
<img src="https://mdbootstrap.com/img/Photos/Horizontal/Nature/8-col/img (132).webp" class="img-fluid" alt="Image of ballons flying over canyons with mask pattern one." />
<MDBMask className="flex-center" pattern={8} overlay="red-strong" >
<p className="white-text">Strong overlay</p>
</MDBMask>
</MDBView>
</MDBContainer>
);
}
export default HoverPage;
Overlay
.webp)
strong overlay
import React from "react";
import { MDBMask, MDBView, MDBContainer } from "mdbreact";
const HoverPage = () => {
return (
<MDBContainer className="mt-5">
<MDBView>
<img src="https://mdbootstrap.com/img/Photos/Others/nature-sm.webp" class="img-fluid" alt="sample image" />
<MDBMask className="flex-center" overlay="green-strong" >
<p className="white-text">text</p>
</MDBMask>
</MDBView>
</MDBContainer>
);
}
export default HoverPage;
Patterns
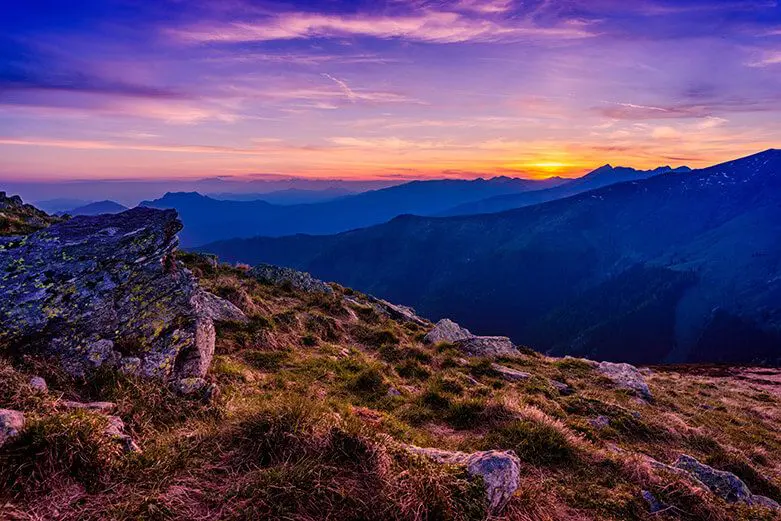
no mask
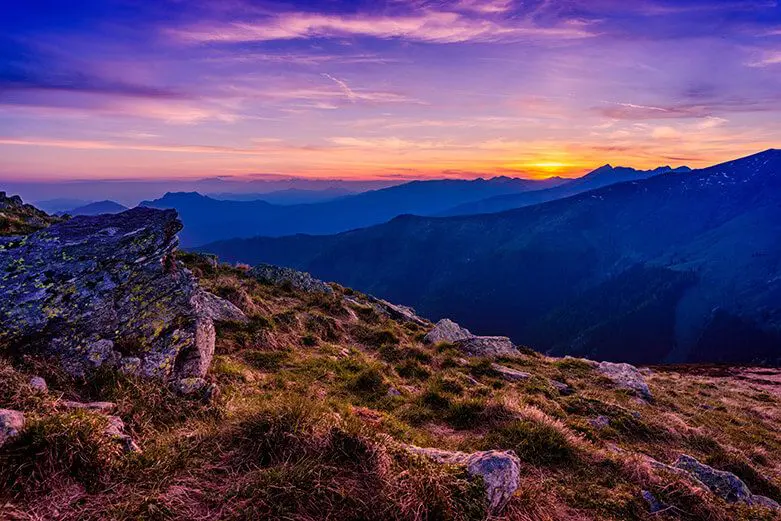
pattern = 1
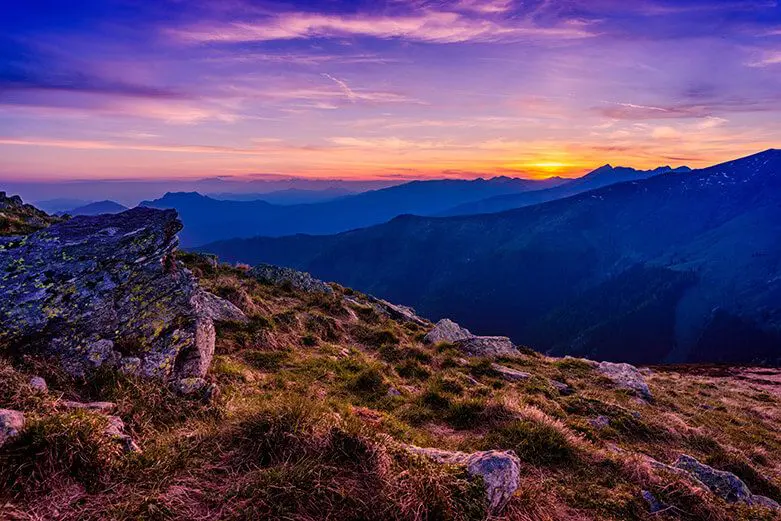
pattern = 2
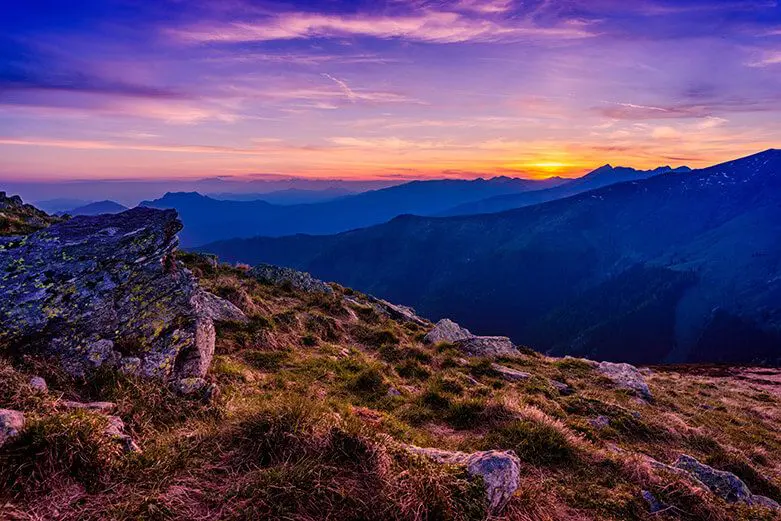
pattern = 3
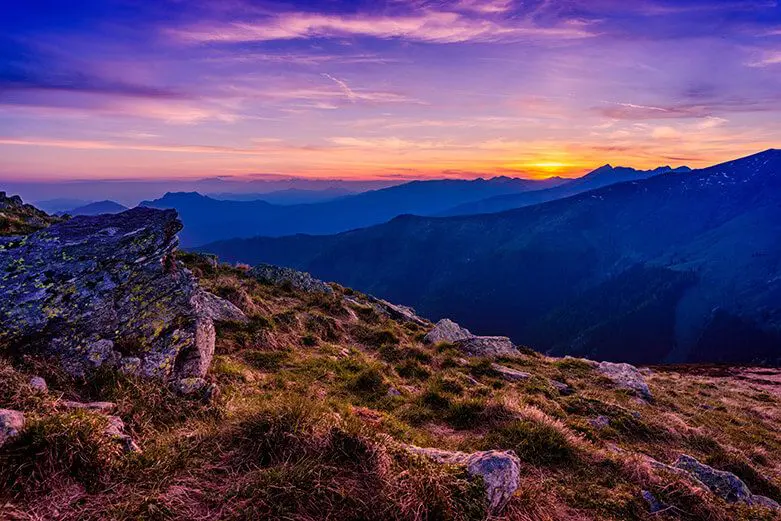
pattern = 4
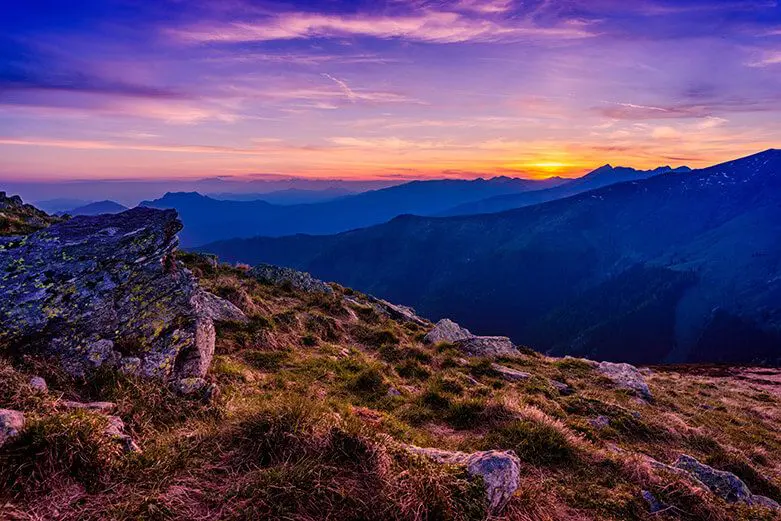
pattern = 5
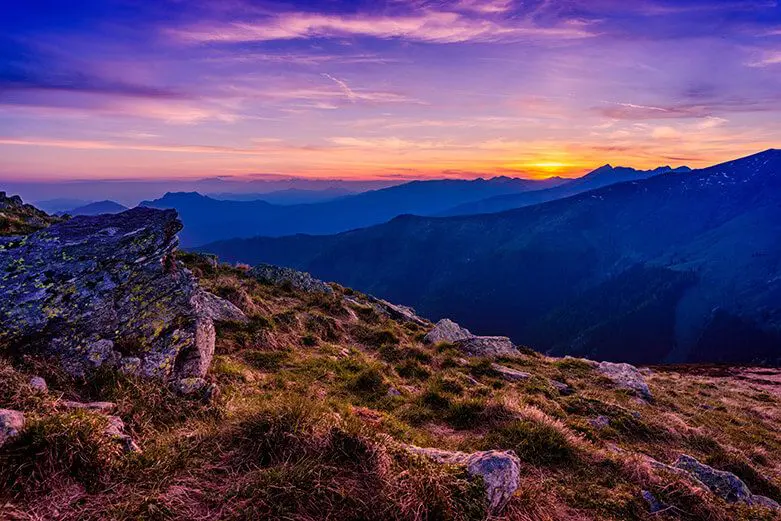
pattern = 6
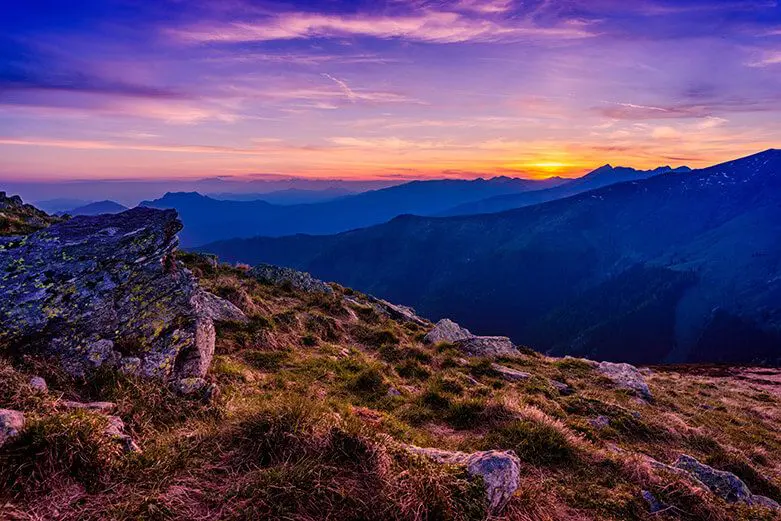
pattern = 7
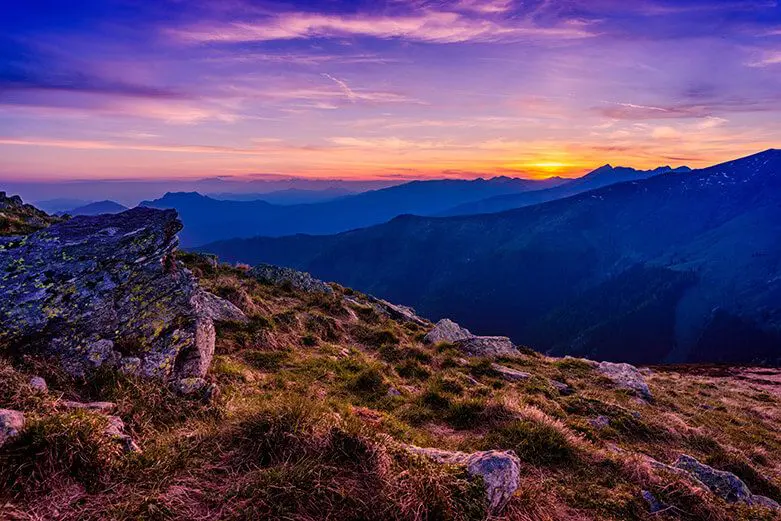
pattern = 8
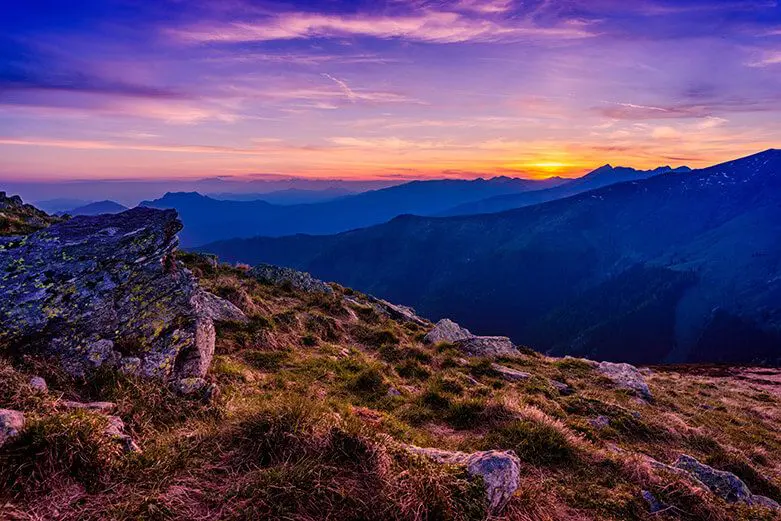
pattern = 9
Usage
Step 1: Create a wrapper containing component <MDBView>
.
<MDBView>
...
</MDBView>
Step 2: Set a path to the image by adding src
attribute.
<MDBView>
<img
src="https://mdbootstrap.com/img/Others/documentation/img%20(131)-mini.webp"
className="img-fluid"
alt=""
/>
</MDBView>
Step 3: Add a component <MDBMask>
to cover the image with the pattern.
<MDBView>
<img
src="https://mdbootstrap.com/img/Others/documentation/img%20(131)-mini.webp"
className="img-fluid"
alt=""
/>
<MDBMask>
</MDBMask>
</MDBView>
Step 4: Choose a pattern prop from the examples above.
<MDBView>
<img
src="https://mdbootstrap.com/img/Others/documentation/img%20(131)-mini.webp"
className="img-fluid"
alt=""
/>
<MDBMask pattern={8}>
</MDBMask>
</MDBView>
Step 5: If you want to add some text, you can use a className flex-center
to center
it.
<MDBView>
<img
src="https://mdbootstrap.com/img/Others/documentation/img%20(131)-mini.webp"
className="img-fluid"
alt=""
/>
<MDBMask pattern={8} className="flex-center">
<p className="white-text">Super light overlay</p>
</MDBMask>
</MDBView>
Overlays
.webp)
slight overlay
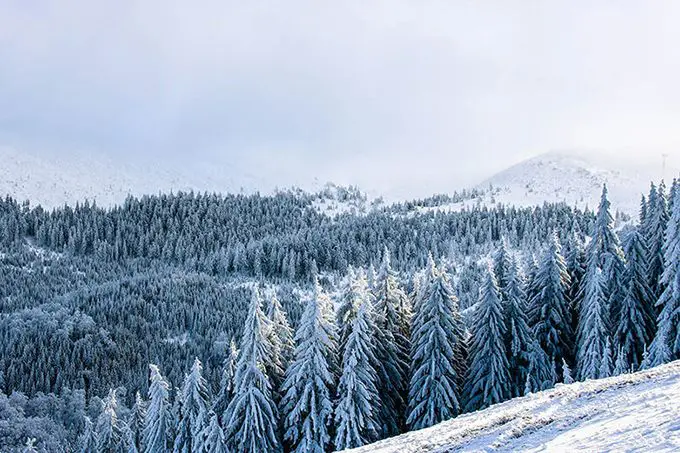
light overlay
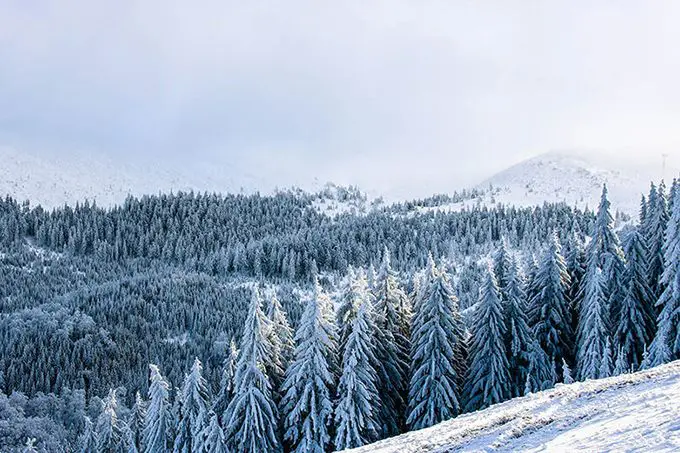
strong overlay
Usage
Instruction and markup are the same like for the patterns. It means you add an overlay prop
(black-strong
in the example below) to the <MDBMask>
.
See the full list of the overlay colors and intensity
<MDBView>
<img
src="https://mdbootstrap.com/img/Others/documentation/img%20(131)-mini.webp"
className="img-fluid"
alt=""
/>
<MDBMask overlay="black-strong" className="flex-center">
<p className="white-text">strong overlay</p>
</MDBMask>
</MDBView>
Overlay colors and intensity
Choose one of the overlay props from the list below to set a desired intensity and color of your mask.
blue-light
red-light
pink-light
purple-light
indigo-light
cyan-light
teal-light
green-light
lime-light
yellow-light
orange-light
brown-light
grey-light
blue-grey-light
black-light
stylish-light
white-light
blue-strong
red-strong
pink-strong
purple-strong
indigo-strong
cyan-strong
teal-strong
green-strong
lime-strong
yellow-strong
orange-strong
brown-strong
grey-strong
blue-grey-strong
black-strong
stylish-strong
white-strong
blue-slight
red-slight
pink-slight
purple-slight
indigo-slight
cyan-slight
teal-slight
green-slight
lime-slight
yellow-slight
orange-slight
brown-slight
grey-slight
blue-grey-slight
black-slight
stylish-slight
white-slight
React Masks - API
In this section you will find advanced information about the Mask component. You will learn which modules are required in this component, what are the possibilities of configuring the component, and what events and methods you can use in working with it.
Import statement
In order to use Hover Masks make sure you have imported proper modules first.
import { MDBView, MDBMask } from "mdbreact";
API Reference: View Properties
The table below shows the configuration options of the MDBView component.
Name | Type | Default | Description | Example |
---|---|---|---|---|
cascade |
Boolean | false |
Places content cascade | <MDBView cascade /> |
className |
String |
|
Adds custom classes | <MDBView className="customClass" /> |
hover |
Boolean | false |
Switches on hover effects | <MDBView hover /> |
rounded |
Boolean | false |
Sets View's border-radius to 50% | <MDBView rounded /> |
src |
String |
|
Sets fixed image backround; accepts url to your image | <MDBView src="/assets/image.webp" /> |
tag |
String | div |
Changes default tag | <MDBView tag="span" /> |
waves |
Boolean | false |
Switches on waves effect (on mouse up) | <MDBView waves /> |
zoom |
Boolean | false |
Switch on zomm efect (on hover) | <MDBView zoom /> |
API Reference: Mask Properties
The table below shows the configuration options of the MDBMask component.
Name | Type | Default | Description | Example |
---|---|---|---|---|
className |
String |
|
Adds custom classes | <MDBMask className="customClass" /> |
overlay |
String |
|
Creates overlay in passed color | <MDBMask overlay="grey-light" /> |
pattern |
String/Number |
|
Creates pattern with chosen style | <MDBMask pattern="1" /> |
tag |
String | div |
Changes default tag | <MDBMask tag="span" /> |